반응형
- 정적 파일 다운로드 -
정적 파일이란 응답 요청이 왔을때 별도의 처리를 하지 않고 바로 보내줄 수 있는 파일들을 말한다.
보통 CSS, HTML, JS 등과 홈페이지의 소개서.pdf, 사용설명서.pdf 등의 파일을 말한다.
1. 코드
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.io.Resource;
import org.springframework.core.io.ResourceLoader;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping("/static-file")
public class StaticFileContoller {
@Autowired
ResourceLoader resourceLoader;
@GetMapping("/{fileName}")
public ResponseEntity<Resource> resouceFileDownload(@PathVariable String fileName) {
try {
Resource resource = resourceLoader.getResource("classpath:static/files/"+ fileName);
File file = resource.getFile(); //파일이 없는 경우 fileNotFoundException error가 난다.
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION,file.getName()) //다운 받아지는 파일 명 설정
.header(HttpHeaders.CONTENT_LENGTH, String.valueOf(file.length())) //파일 사이즈 설정
.header(HttpHeaders.CONTENT_TYPE, MediaType.APPLICATION_OCTET_STREAM.toString()) //바이너리 데이터로 받아오기 설정
.body(resource); //파일 넘기기
} catch (FileNotFoundException e) {
e.printStackTrace();
return ResponseEntity.badRequest()
.body(null);
} catch (Exception e ) {
e.printStackTrace();
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
}
위에 코드에서 보면 /static-file 이라는 RequestMapping을 주고 fileName 이라는 URL 패스로 파일을 다운로드 하게 만들었다.
ResponseEntity 를 사용하여 resource 를 바디에 담아주며 헤더에 다운받아지는 파일 명, 파일 사이즈, 콘텐트 타입등을 설정해서 반환한다.
또한 FileNotFoundException 처리를 해서 만약 url에 주소를 잘못 입력한 경우 badRequest(400 에러)가 응답되도록 구현하였다.
Spring boot 자동 설정에 따른 classpath 중 하나인 /src/main/resources 아래의 files/파일명.확장자로 파일을 다운 찾도록 하였다.
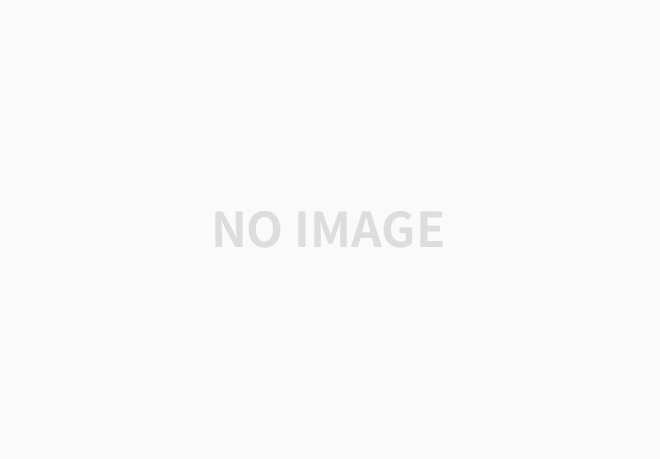
반응형
'Spring > spring boot' 카테고리의 다른 글
@ConfigurationProperties 사용법 - 삽질중인 개발자 (0) | 2020.06.01 |
---|---|
Spring boot 로그 설정 및 출력 방법- logback 설정하기 - 삽질중인 개발자 (0) | 2020.05.24 |
Spring boot oracle db 연동 , spring boot oracle jdbc 설정 - 삽질중인 개발자 (0) | 2019.12.16 |
스프링 부트 JSP 연동, Spring boot MVC 설정 방법 - 삽질중인 개발자 (0) | 2019.12.16 |
Spring boot tomcat 설정, 스프링 부트 톰캣 포트 설정 - 삽질중인 개발자 (0) | 2019.12.16 |